Mastering Jetpack Compose
Master the art of modern UI development with Jetpack Compose for Android and multiplatform applications.
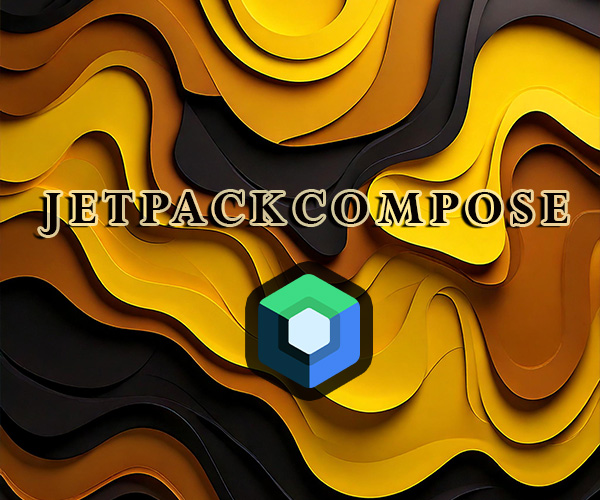
Book Contents
Core Concepts
Chapters 1-6: Master the fundamentals of Jetpack Compose and multiplatform development.
- Development environment setup
- Declarative UI principles
- State management
UI Development
Chapters 7-14: Advanced UI components, animations, and user interaction.
- Custom components
- Complex animations
- Gesture handling
Real-world Applications
Chapters 15-20: Production-ready applications and best practices.
- Performance optimization
- Platform-specific features
- Complete applications
- • Understanding the Kotlin Multiplatform Ecosystem
- • Development Environment Setup
- • Project Configuration
- • Project Structure Deep Dive
- • Getting Started with Development
- • Understanding the Declarative UI Paradigm
- • Core Concepts of Compose
- • Compose vs Traditional UI Development
- • Compose Syntax and Patterns
- • Building Your First Compose Screen
- • Understanding Shared Code Architecture
- • Platform-Specific Implementations
- • Dependency Management
- • Code Organization Strategies
- • Common Multiplatform Patterns
- • Text and Typography
- • Interactive Elements
- • Visual Elements
- • Collection Components
- • Input Components
- • Navigation Components
- • Custom Components
- • Understanding the Compose Layout System
- • Core Layout Composables
- • ConstraintLayout in Compose
- • Custom Layouts
- • Responsive Design Patterns
- • Platform-Specific Considerations
- • Understanding Design Systems
- • Color Management
- • Typography System
- • Dark Mode Implementation
- • Platform-Specific Styling
- • Custom Theme Implementation
- • Material Design 3 Integration
- • Understanding Animation Fundamentals
- • Basic Animation Implementation
- • Complex Transition Effects
- • Custom Animation Development
- • Canvas Drawing and Graphics
- • Graphics Optimization
- • Platform-Specific Implementation
- • Understanding Input Fundamentals
- • Basic Touch and Click Handling
- • Drag and Drop Implementation
- • Swipe Gesture Systems
- • Multi-touch Implementation
- • Platform-Specific Input Patterns
- • Custom Gesture Recognition
- • Understanding Navigation Architecture
- • Implementing Deep Linking
- • Screen Transition Management
- • State Preservation Systems
- • Platform Navigation Patterns
- • Custom Navigation Solutions
- • Understanding State in Compose
- • ViewModel Architecture
- • State Hoisting Implementation
- • Side Effects Management
- • Platform-Specific State Management
- • State Restoration
- • Understanding Kotlin Flow in Compose
- • Repository Pattern Implementation
- • Dependency Injection Systems
- • Clean Architecture Implementation
- • Platform-Specific Architecture
- • Cross-Platform Data Sharing
- • Modern Key-Value Storage Solutions
- • SQLite Database Implementation
- • File System Management
- • Caching Implementation
- • Platform-Specific Storage
- • Data Migration
- • HTTP Client Architecture
- • API Service Architecture
- • Error Handling Systems
- • Response Processing
- • Authentication Implementation
- • Offline Support Implementation
- • WebSocket Communication
- • Push Notification Systems
- • Background Processing
- • Platform-Specific Networking
- • Real-time Data Synchronization
- • Network Security
- • Social Media Integration
- • Payment Gateway Integration
- • Location Services Implementation
- • Analytics Implementation
- • Crash Reporting Systems
- • Platform-Specific Service Integration
- • Understanding Compose Performance
- • Memory Management
- • UI Rendering Optimization
- • Network Performance
- • Image Loading and Caching
- • Platform-Specific Optimization
- • Designing Loading States
- • Error Handling and User Communication
- • Offline Experience Design
- • Accessibility Implementation
- • Localization Systems
- • Platform-Specific UX Patterns
- • Custom Rendering Implementation
- • Complex Interaction Systems
- • Advanced Animation Systems
- • Platform Integration
- • Security Implementation
- • App Distribution and Deployment
- • Project Planning and Foundation
- • Implementation Strategy
- • Feature Development Process
- • Platform-Specific Implementation
- • Quality Assurance Implementation
- • Deployment Preparation
- • iOS Platform Integration
- • Android Platform Integration
- • Desktop Platform Considerations
- • Platform UI Guidelines
- • Hardware Integration
- • Platform Distribution
- • Advanced Composables Pattern Library
- • Material Design 3 Component Reference
- • Performance Optimization Techniques
- • Cross-platform Implementation Guidelines
- • Common Issues and Solutions
- • Third-party Libraries and Tools
- • Migration Strategies
- • Testing Approaches and Tools
- • Deployment Checklists
- • Resource Links and Documentation
What's Included
500+ Code Examples
Comprehensive, production-ready examples covering everything from basic UI components to complex animations and state management.
Cross-Platform Projects
Build complete multiplatform applications targeting Android, iOS, and Desktop platforms using Compose.
Free Updates
Stay current with the latest Jetpack Compose features and best practices as the framework evolves.
What You'll Learn
Technical Skills
Master declarative UI development with Jetpack Compose
Build apps that work seamlessly across Android, iOS, and Desktop
Create complex, interactive animations and transitions
Implement robust state management solutions
Professional Skills
Build high-performance, responsive applications
Create beautiful, consistent user interfaces
Implement comprehensive testing solutions
Design scalable, maintainable applications
Start Your Jetpack Compose Journey Today
Join the next generation of app developers building beautiful, native applications with Jetpack Compose.
Choose Your PlanStay Updated
Get access to new content and examples as Jetpack Compose evolves. All future updates are delivered via email.
- New content additions
- Latest feature coverage
- Additional examples